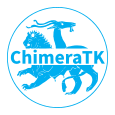 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
4 #include "../NDRegisterAccessor.h"
5 #include "../RegisterPath.h"
53 static std::unique_ptr<GenericMuxedInterruptDistributor>
create(std::string
const& description,
62 boost::shared_ptr<NDRegisterAccessor<uint32_t>>
_isr;
63 boost::shared_ptr<NDRegisterAccessor<uint32_t>>
_ier;
64 boost::shared_ptr<NDRegisterAccessor<uint32_t>>
_icr;
65 boost::shared_ptr<NDRegisterAccessor<uint32_t>>
_mer;
67 boost::shared_ptr<NDRegisterAccessor<uint32_t>>
_sie;
68 boost::shared_ptr<NDRegisterAccessor<uint32_t>>
_cie;
void clearAllEnabledInterrupts()
boost::shared_ptr< NDRegisterAccessor< uint32_t > > _mer
Send backend-specific asynchronous data to different distributors:
uint32_t _activeInterrupts
boost::shared_ptr< NDRegisterAccessor< uint32_t > > _icr
void clearOneInterrupt(uint32_t ithInterrupt)
GenericMuxedInterruptDistributor(const boost::shared_ptr< SubDomain< std::nullptr_t >> &parent, const std::string ®isterPath, std::bitset<(ulong) GmidOptionCode::OPTION_CODE_COUNT > optionRegisterSettings)
void clearInterruptsFromMask(uint32_t mask)
In mask, 1 bits clear the corresponding registers, 0 bits do nothing.
void activateSubDomain(SubDomain< std::nullptr_t > &subDomain, VersionNumber const &version) override
Function to activate a (new) single SubDomain if the MuxedInterruptDistributor is already active.
void enableInterruptsFromMask(uint32_t mask)
For each bit in mask that is a 1, the corresponding interrupt gets enabled, and the internal copy of ...
boost::shared_ptr< NDRegisterAccessor< uint32_t > > _cie
boost::shared_ptr< NDRegisterAccessor< uint32_t > > _ier
static std::unique_ptr< GenericMuxedInterruptDistributor > create(std::string const &description, const boost::shared_ptr< SubDomain< std::nullptr_t >> &parent)
Create parses the json configuration snippet 'description', and calls the constructor.
void enableOneInterrupt(uint32_t ithInterrupt)
~GenericMuxedInterruptDistributor() override
void activate(VersionNumber version) override
void clearAllInterrupts()
void handle(VersionNumber version) override
Handle gets called when a trigger comes in.
Interface base class for interrupt controller handlers.
boost::shared_ptr< NDRegisterAccessor< uint32_t > > _sie
boost::shared_ptr< NDRegisterAccessor< uint32_t > > _isr
Class to store a register path name.
Class for generating and holding version numbers without exposing a numeric representation.
void disableOneInterrupt(uint32_t ithInterrupt)
void disableInterruptsFromMask(uint32_t mask)
Disables each interrupt corresponding to the 1 bits in mask, and updates _activeInterrupts.